Python is
Features(advantages):
Limitations
As compared to the popular technologies like JDBC and ODBC, the Python’s database access layer is found to be bit underdeveloped and primitive.
Working in Python: You can work in python using two modes.
1.Interactive mode(immediate mode): commands are given in front of Python prompt( >>>) one at a time. Then python executes the given command and give you output. For example
>>>2+5
7
Steps:
click on start button
Click on all programs
Click on python3.7
Then click on python3.7 it will display command prompt(python shell) as
>>>
Type your command and press enter
>>>2+5
7
We cannot save the commands in Interactive mode.
1.Script mode(Python IDLE): Python instructions or commands can be store in a file or script with .py extension and the file can be executed in one go as a unit. This saved file is called python script or python program.
Steps:
(A) Create program file
print(“Hello World”)
4. File->Save and save file with a name hello.py and click on save button
(B) Run program file:
Hello World
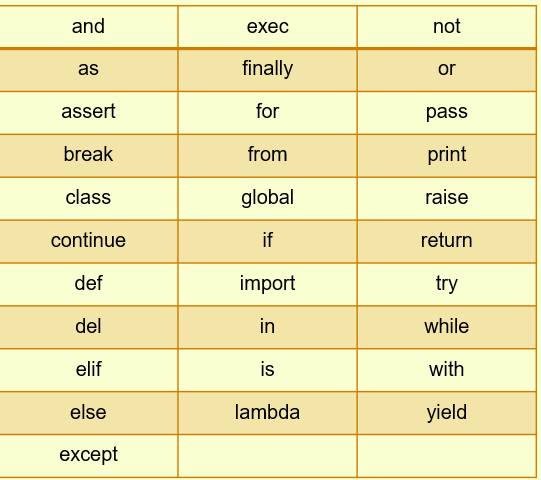
Literals(Constants-Values):
S=‘hello\
how are\
you’
>>>S
Hello how are you
(b) By typing text in triple quotation marks(‘’’ ‘’’)
for example
>>>s=‘’’ hello
how are
you’’’
>>>print(s)
hello
how are
you
Escape Characters
An escape character is a backslash \ followed by the character you want to insert.
#This example erases one character (backspace):
txt = "Hello \bWorld!"
print(txt)
Output :HelloWorld
#A backslash followed by three integers will result in a octal value:
txt = "\110\145\154\154\157"
print(txt)
2.Numeric literals:
(a) integers (b) float
Integers: numbers without fractional(decimal point) part. May contain +or – sign.
Float(real):numbers with fractional parts. May contain +or – sign. Must contain decimal point(.)
For example 0x12FCD, 0X4A5EF, 0xD etc.
Long Integers:
Integers of unlimited size followed by lowercase or uppercase L eg: 87032845L
Complex numbers:
In the form of a+bj where a forms the real part and b forms the imaginary part of the complex number. eg: 3.14j
Float literals: they may written in two forms
mantissa E exponent
For example 5.8 can be written as
0.58 * 101=0.58E01 where mantissa is 0.58 and 01 is exponent
NOTE* numeric values with commas are not considered int or float value , rather Python treats them as a tuple. A tuple is a special type in Python that stores a sequences of values
3. None Literal: It is used to indicate absence of value.
>>>a=None
>>>a
>>>print(a)
4. Boolean literals : True and False are two Boolean literal in Python.Logical operators always results in True or False
>>>a=10
>>>b=25
>>>a>b
False
5.Literal Collections
Python provides the four types of literal collections
List, tuple,Set, Dictionary
List:
List contains items of different data types. Lists are mutable i.e., modifiable.The values stored in List are separated by comma(,) and enclosed within square brackets([]). We can store different types of data in a List.
list=[1,2,3,4]
Tuple:
Python tuple is a collection of different data-type. It is immutable which means it cannot be modified after creation.It is enclosed by the parentheses () and each element is separated by the comma(,)
list=(1,2,3,4)
Set:
Python set is the collection of the unordered dataset.It is enclosed by the {} and each element is separated by the comma(,).
set = {'apple','grapes','guava','papaya'}
print(set)
Dictionary:
Python dictionary stores the data in the key-value pair.
It is enclosed by curly-braces {} and each pair is separated by the commas(,).
dict = {'name': 'Pater', 'Age':18,'Roll_nu':101}
print(dict)
Operators:
Operators can be defined as symbols that are used to perform
arithmetic and logical operations on data or variable called operands.
Types of Operators
1. Arithmetic Operators.
2. Relational(comparison) Operators.
3. Assignment Operators.
4. Logical Operators.
5. Bitwise Operators
6. Membership Operators
7. Identity Operators
Arithmetic Operators
Arithmetic operators are used to perform mathematical operations like addition, subtraction, multiplication, etc.
+,-,*,
** (Exponentiation) Exponent - left operand raised to the power of right ,
/ (division )Divide left operand by the right one (always results into float) ,
// ( Floor division )Modulus - remainder of the division of left operand by the right ,
% (Modulus)
x = 15
y = 4
# Output: x + y = 19
print('x + y =',x+y)
# Output: x - y = 11
print('x - y =',x-y)
# Output: x * y = 60
print('x * y =',x*y)
# Output: x / y = 3.75
print('x / y =',x/y)
# Output: x // y = 3
print('x // y =',x//y)
# Output: x ** y = 50625
print('x ** y =',x**y)
Relational Operators
Comparison operators are used to compare values. It returns either True or False according to the condition.
These are >,>=,<.<=.==,!=
x = 10
y = 12
# Output: x > y is False
print('x > y is',x>y)
# Output: x < y is True
print('x < y is',x<y)
# Output: x == y is False
print('x == y is',x==y)
# Output: x != y is True
print('x != y is',x!=y)
# Output: x >= y is False
print('x >= y is',x>=y)
# Output: x <= y is True
print('x <= y is',x<=y)
Assignment Operators(=)
Assignment operators are used in Python to assign values to variables.
a = 5 is a simple assignment operator that assigns the value 5 on the right to the variable a on the left.
There are various (shorthand Assignment Operators) compound operators in Python like a += 5 that adds to the variable and later assigns the same. It is equivalent to a = a + 5.
These are +=,-=,*=,/=, //=,%=, **=,
a=5
a+=5 equals a=a+5
a-=5 equals a=a-5
a*=5 equals a=a*5
Logical operators
and ,or and not operators are logical operators. Always returns true or false
and returns true if both operands/conditions are true
or returns true if either of the operand is true
not returns false if operand is true
x = True
y = False
print('x and y is',x and y) # False
print('x or y is',x or y) # True
print('not x is',not x) #False
Membership Operators
in and not in are the membership operators in Python. They are used to test whether a value or variable is found in a sequence (string, list, tuple, set and dictionary).
x = 'Hello world'
y = {1:'a',2:'b'}
# Output: True
print('H' in x)
# Output: True
print('hello' not in x)
# Output: True
print(1 in y)
# Output: False
print('a' in y)
Identity operators
is and is not are the identity operators in Python. They are used to check if two values (or variables) are located on the same part of the memory. It returns true if both have same object id. Two variables that are equal does not imply that they are identical.
When you ask Python about whether one object is the same as another object, you are asking if they have the same identity.
x1 = 5
y1 = 5
x2 = 'Hello'
y2 = 'Hello'
x3 = [1,2,3]
y3 = [1,2,3]
# Output: False
print(id(x2)," ", id(y1))
print(x1 is not y1) # compare object Identity not values
# Output: True
print(x2 is y2)
# Output: False
print(x3 is y3)
NOTE
The == operator compares the value or equality of two objects, whereas the Python is operator checks whether two variables point to the same object in memory.
IS compare the reference of two variable is same or not while == operator compare values of two variables.
>>> s1='abc'
>>> s2='abc'
>>> s1==s2 #compare values of s1 and s2
True
>>> s1 is s2 # compare reference of s1 and s2
True
e,g
Python create two different object with same value when:
input string from the keyboard/Assign complex values/
>>> s2=input("Enter a string")
Enter a string abc
>>> s1 is s2 # python create two different object with same value
False
e.g
>>> a=2+3.5j
>>> b=2+3.5j
>>> a is b
False
>>> a==b
True
Bitwise operators
Bitwise operators are used to compare (binary) numbers:
For example, 2 is 10 in binary and 7 is 111
& (AND) Sets each bit to 1 if both bits are 1
|(OR) Sets each bit to 1 if one of two bits is 1
^(XOR) Sets each bit to 1 if only one of two bits is 1
~( NOT) Inverts all the bits
<< ( Zero fill left shift )
shifts the left operand bits towards the left side for the given number of times in the right operand. In simple terms, the binary number is appended with 0s at the end.
Shift left by pushing zeros in from the right and let the leftmost bits fall off
>> (Signed right shift) Shift right by pushing copies of the leftmost bit in from the left, and let the rightmost bits fall off
Let x = 10 (0000 1010 in binary) and y = 4 (0000 0100 in binary)
Operator Meaning Example
& Bitwise AND x & y = 0 (0000 0000)
| Bitwise OR x | y = 14 (0000 1110)
~ Bitwise NOT ~x = -11 (1111 0101)
^ Bitwise XOR x ^ y = 14 (0000 1110)
>> Bitwise right shift x >> 2 = 2 (0000 0010)
<< Bitwise left shift x << 2 = 40 (0010 1000)
Operator Precedence
When an expression involve multiple operators , python resolves the order of execution through operator precedence.
Operator Associativity
When two operators have the same precedence, associativity helps to determine which the order of operations.
Associativity is the order in which an expression is evaluated that has multiple operator of the same precedence. Almost all the operators have left-to-right associativity.
For example, multiplication and floor division have the same precedence. Hence, if both of them are present in an expression, left one is evaluates first.
# Left-right associativity
# Output: 3
print(5 * 2 // 3)
# Shows left-right associativity
# Output: 0
print(5 * (2 // 3))
**(RIGHT TO LEFT ASSOCIATIVITY)
>>> 2**3**2
512
>>>2**2**3
256
Variable :
Variable:
Variables are nothing but reserved memory locations. This means that when you create a variable you reserve some space in memory.
x = 5
y = "John"
print(x)
print(y)
x = 4 # x is of type int
x = "Sally" # x is now of type str
print(x)
Multiple Assignment
Python allows you to assign a single value to several variables simultaneously. For example −
a = b = c = 1
Here, an integer object is created with the value 1, and all three variables are assigned to the same memory location. You can also assign multiple objects to multiple variables. For example −
a,b,c = 1,2,"john"
Here, two integer objects with values 1 and 2 are assigned to variables a and b respectively, and one string object with the value "john" is assigned to the variable c.
if we assign multiple values to a single variable
a=1,2,3
print(a) #a will be tread by default tuple
ouput
(1, 2, 3)
Naming variable :
Print variable : using print() method
Scope of variable : local and global
Data type
Standard Data Types
The data stored in memory can be of many types. For example, a person's age is stored as a numeric value and his or her address is stored as alphanumeric characters. Python has various standard data types that are used to define the operations possible on them and the storage method for each of them.
Python data types −
Numbers (int,float,complex)
boolean
String
None
Sequences (List,Tuple,set,Dictionary)
Number In Python
It is used to store numeric values Python has three numeric types: 1. Integers 2. Floating point numbers 3. Complex numbers
3. Complex numbers
Complex numbers are combination of a real and imaginary part.Complex numbers are in the form of X+Yj, where X is a real part and Y is imaginary part.
e.g.
a = complex(5)
# convert 5 to a real part val and zero imaginary part print(a)
b=complex(101,23)
#convert 101 with real part and 23 as imaginary part
print(b)
Code Output :-
(5+0j) (101+23j)
Comments in Python
which is readable for programmer but ignored by python interpreter
i. Single line comment: Which begins with # sign.
ii. Multi line comment (docstring): either write multiple line beginning with #
sign or use triple quoted multiple line. E.g.
‘’’this is my
first
python multiline comment
‘’’
List
fruits = ["apple", "banana", "cherry"]
for x in fruits:
print(x)Change Item Value
To change the value of a specific item, refer to the index number:
fruit = ["apple", "banana", "cherry"]
thislist[1] = "blackcurrant"
print(thislist)
for loop :
# Program to find the sum of all numbers stored in a list
# List of numbers
numbers = [6, 5, 3, 8, 4, 2, 5, 4, 11]
# variable to store the sum
sum = 0
#iterate over the list
for val in numbers:
sum = sum+val
# Output:
The sum is 48print("The sum is", sum)
For loop with else clause
else block will be executed if loop terminated normally
digits = [0, 1, 5]
for i in digits:
print(i)
else:
print("No items left.")
Otherwise else block will not executed
Comments
Post a Comment